Mastering PHP: Advanced Assignments and Solutions
Welcome, fellow programmers and enthusiasts, to programminghomeworkhelp.com – your ultimate destination for mastering PHP and excelling in your assignments! As a dedicated PHP assignment helper, our mission is to equip you with the knowledge and skills needed to tackle even the most challenging tasks in PHP programming.
Today, we delve into the realm of advanced PHP assignments, where intricate problem-solving and creative solutions are paramount. Through this post, we'll explore two master-level PHP questions along with comprehensive solutions crafted by our expert. So, without further ado, let's dive in!
Question 1: Dynamic Content Generation
You've been tasked with developing a dynamic content generation system for a news website. The system should fetch articles from a database and display them on the homepage. Each article should include its title, publication date, author, and a brief excerpt. Additionally, the homepage should feature pagination to navigate through multiple pages of articles, with each page displaying a specified number of articles.
Solution:
<?php
// Establish database connection
$servername = "localhost";
$username = "username";
$password = "password";
$dbname = "news_database";
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Pagination variables
$articles_per_page = 10;
$page = isset($_GET['page']) ? $_GET['page'] : 1;
$start = ($page - 1) * $articles_per_page;
// Fetch articles from database
$sql = "SELECT * FROM articles LIMIT $start, $articles_per_page";
$result = $conn->query($sql);
// Display articles
if ($result->num_rows > {
while($row = $result->fetch_assoc()) {
echo "<h2>" . $row["title"] . "</h2>";
echo "<p>Published on: " . $row["publication_date"] . " by " . $row["author"] . "</p>";
echo "<p>" . substr($row["content"], 0, 15 . "...</p>"; // Display excerpt
}
} else {
echo "No articles found";
}
// Pagination links
$sql = "SELECT COUNT(*) AS total FROM articles";
$result = $conn->query($sql);
$row = $result->fetch_assoc();
$total_articles = $row["total"];
$total_pages = ceil($total_articles / $articles_per_page);
echo "<div>";
for ($i = 1; $i <= $total_pages; $i++) {
echo "<a href='?page=$i'>$i</a> ";
}
echo "</div>";
// Close connection
$conn->close();
?>
Question 2: Secure User Authentication
You're building a web application that requires user authentication to access certain features. Implement a secure login system using PHP and MySQL. The system should verify the user's credentials against those stored in the database and grant access only to authenticated users.
Solution:
<?php
session_start();
// Establish database connection
$servername = "localhost";
$username = "username";
$password = "password";
$dbname = "user_database";
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Login function
function login($username, $password, $conn) {
$username = mysqli_real_escape_string($conn, $username);
$password = mysqli_real_escape_string($conn, $password);
$sql = "SELECT * FROM users WHERE username='$username' AND password='$password'";
$result = $conn->query($sql);
if ($result->num_rows == 1) {
$_SESSION['username'] = $username;
header("Location: dashboard.php" // Redirect to dashboard upon successful login
exit();
} else {
echo "Invalid username or password";
}
}
// Logout function
function logout() {
session_unset();
session_destroy();
header("Location: index.php" // Redirect to login page upon logout
exit();
}
// Logout if logout button is clicked
if (isset($_GET['logout'])) {
logout();
}
// Check if the login form is submitted
if ($_SERVER["REQUEST_METHOD"] == "POST" {
if (isset($_POST['username']) && isset($_POST['password'])) {
$username = $_POST['username'];
$password = $_POST['password'];
login($username, $password, $conn);
}
}
?>
<!-- Login Form -->
<form method="post" action="<?php echo htmlspecialchars($_SERVER["PHP_SELF"]);?>">
<label for="username">Username:</label>
<input type="text" name="username" required><br><br>
<label for="password">Password:</label>
<input type="password" name="password" required><br><br>
<button type="submit">Login</button>
</form>
<!-- Logout Button -->
<a href="?logout=true">Logout</a>
In conclusion, mastering PHP involves not only understanding the syntax but also applying it to solve real-world problems effectively. We hope these master-level PHP questions and solutions have provided valuable insights and enhanced your PHP programming skills. Remember, practice makes perfect, so keep coding and exploring the endless possibilities of PHP! Stay tuned for more expert guidance and assistance from https://www.programminghomeworkhelp.com/php/.
#phpassignmenthelp #phpassignmenthelper #phpassignment #programmingassignmenthelp #programmingassignment #assignmenthelp #education #students #university #college
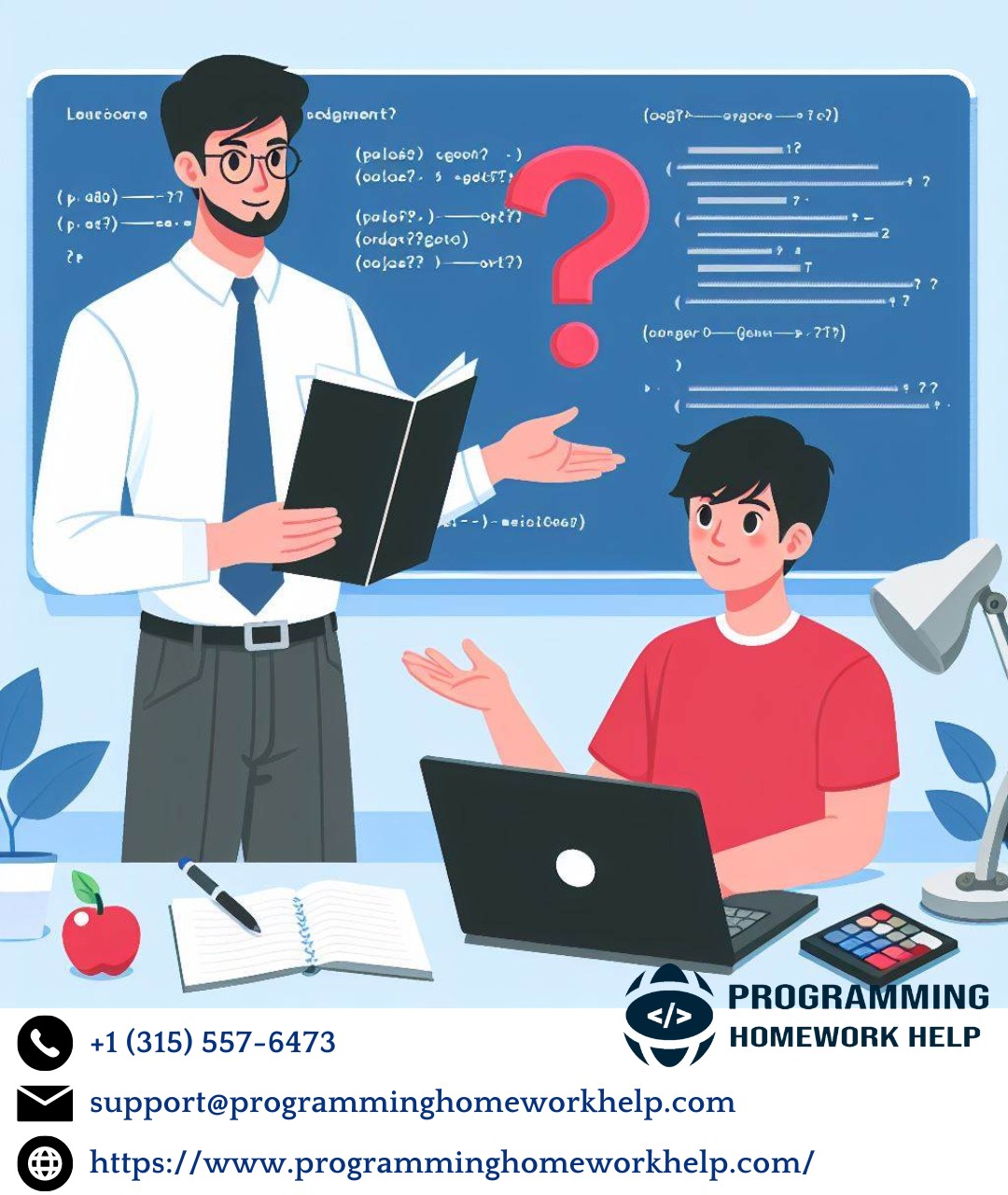